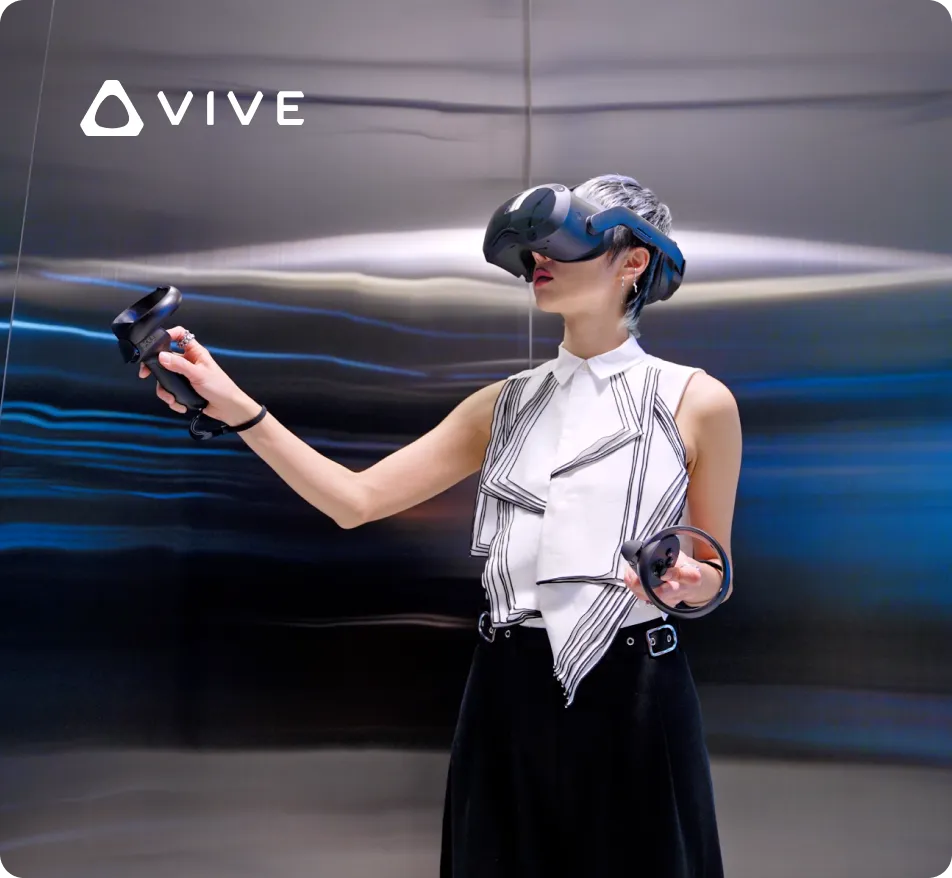

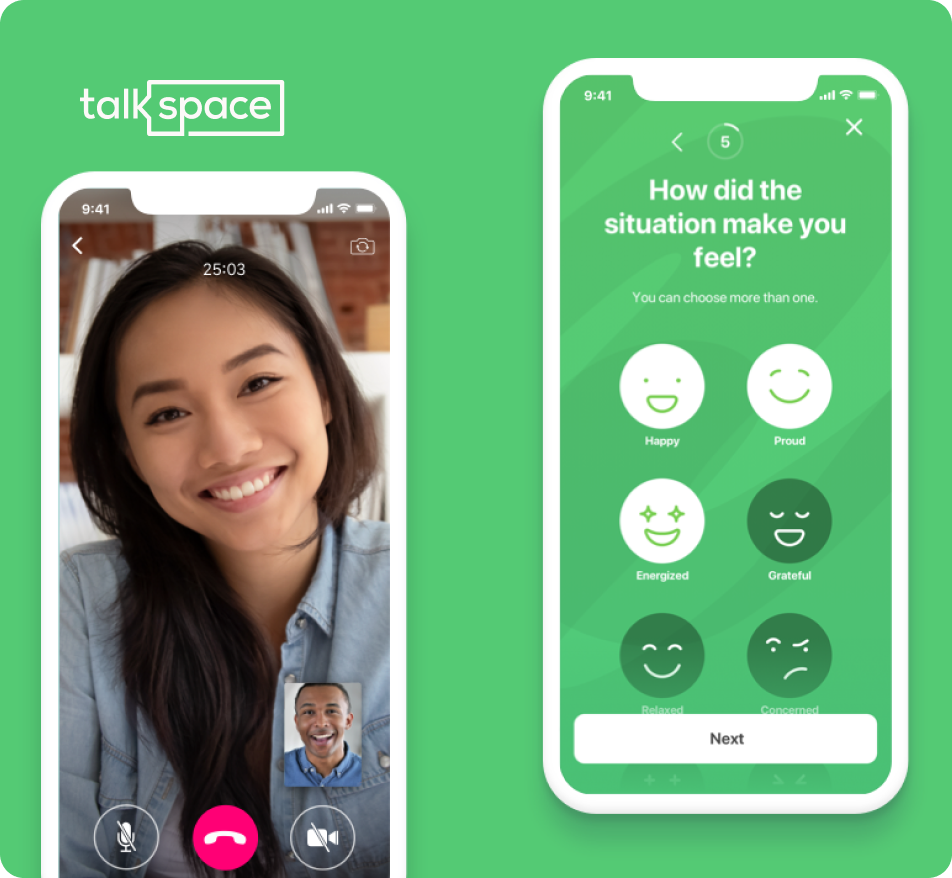
1RtcEngineConfig config = new RtcEngineConfig();
2config.mAppId = "Your app ID";
3RtcEngine agoraEngine = RtcEngine.create (config);
4agoraEngine.setupLocalVideo(new VideoCanvas(new SurfaceView(getBaseContext(), VideoCanvas.RENDER_MODE_HIDDEN, 0));
5agoraEngine.enableVideo();
6agoraEngine.startPreview();
7agoraEngine.joinChannel("<Your token>", "<Your channel name>", 1, new ChannelMediaOptions());
8agoraEngine.enableVideo();
1config.appId = "<Your app id>"
2agoraEngine = AgoraRtcEngineKit.sharedEngine(with: config, delegate: self)
3option.clientRoleType = .broadcaster
4agoraEngine.enableVideo()
5videoCanvas.view = localView
6agoraEngine.setupLocalVideo(videoCanvas)
7agoraEngine.startPreview()
8agoraEngine. (byToken: "<Your token>", channelId: "<channel name>", uid: 0, mediaOptions: option, joinSuccess: { (channel, uid, elapsed) in })
1IRtcEngine* agoraEngine = createAgoraRtcEngine();
2RtcEngineContext context;
3context.appId = "<Your app ID>";
4context.channelProfile = CHANNEL_PROFILE_LIVE_BROADCASTING;
5agoraEngine->initialize(context);
6agoraEngine->setClientRole(CLIENT_ROLE_TYPE::CLIENT_ROLE_BROADCASTER);
7agoraEngine->setupLocalVideo(<VideoCanvas>);
8agoraEngine->enableVideo();
9agoraEngine->startPreview();
10agoraEngine->joinChannel("Your Token", "<Your channel name>", 0, NULL);
1const agoraEngine = AgoraRTC.createClient({ mode: "rtc", codec: "vp8" });
2agoraEngine.setClientRole("host");
3await agoraEngine.join("<Your app ID>", "<Your channel Name>", "<Your token>", 1);
4var localAudioTrack = await AgoraRTC.createMicrophoneAudioTrack();
5var localVideoTrack = await AgoraRTC.createCameraVideoTrack();
6await agoraEngine.publish([localAudioTrack, localVideoTrack]);
7localVideoTrack.play(document.createElement("div"));
1var agoraEngine = createAgoraRtcEngine();
2agoraEngine.initialize({appId: "<Your app ID>"});
3agoraEngine.setChannelProfile(ChannelProfileType.ChannelProfileCommunication);
4agoraEngine.setClientRole(ClientRoleType.ClientRoleBroadcaster);
5agoraEngine.setupLocalVideo({sourceType: VideoSourceType.VideoSourceCameraPrimary,view: <Pass a div element here>});
6agoraEngine.enableVideo();
7agoraEngine.startPreview();
8agoraEngine.joinChannel("<Your token>", "<You channel name>", 1);
1RtcEngine agoraEngine = createAgoraRtcEngine();
2await agoraEngine.initialize(const RtcEngineContext(appId: "<Your app ID>"));
3ChannelMediaOptions options = const ChannelMediaOptions(clientRoleType: ClientRoleType.clientRoleBroadcaster,
4 channelProfile: ChannelProfileType.channelProfileCommunication);
5<Use a Container here> = AgoraVideoView(controller: VideoViewController(rtcEngine: agoraEngine,canvas: VideoCanvas(uid: 0));
6await agoraEngine.enableVideo();
7await agoraEngine.startPreview();
8await agoraEngine.joinChannel(token: <"You token">,channelId: "You channel name", options: options, uid: 1);
1import { createAgoraRtcEngine, IRtcEngine} from 'react-native-agora';
2const agoraEngine = useRef<IRtcEngine>();
3agoraEngine.initialize({appId: "<Your app ID>",channelProfile: ChannelProfileType.ChannelProfileLiveBroadcasting});
4agoraEngine.setChannelProfile(ChannelProfileType.ChannelProfileCommunication);
5agoraEngine.enableVideo();
6agoraEngine.startPreview();
7agoraEngine.joinChannel("<Your token>", "<You channel name>", 1, {clientRoleType: ClientRoleType.ClientRoleBroadcaster});
1IRtcEngine agoraEngine = Agora.Rtc.RtcEngine.CreateAgoraRtcEngine();
2RtcEngineContext context = new RtcEngineContext("<You app ID>", 0,CHANNEL_PROFILE_TYPE.CHANNEL_PROFILE_COMMUNICATION, "");
3agoraEngine.Initialize(context);
4agoraEngine.SetClientRole(CLIENT_ROLE_TYPE.CLIENT_ROLE_BROADCASTER);
5agoraEngine.EnableVideo();
6VideoSurface LocalView.SetForUser(0, "", VIDEO_SOURCE_TYPE.VIDEO_SOURCE_CAMERA);
7LocalView.SetEnable(true);
8agoraEngine.JoinChannel("Your token", "You channel");
1RtcEngineContext RtcEngineContext;
2RtcEngineContext.appId = <Your AppId>
3RtcEngineContext.eventHandler = new agora::rtc::IRtcEngineEventHandler();
4
5RtcEngineProxy = agora::rtc::ue::createAgoraRtcEngine();
6RtcEngineProxy->initialize(RtcEngineContext);
7
8RtcEngineProxy->enableAudio();
9RtcEngineProxy->enableVideo();
10RtcEngineProxy->joinChannel(<Your Token>, <Your ChannelName>, "", 0);